React¶
React is a javascript library for building reactive user interfaces and it’s Declarative.
design simple views for each state in your application
react will efficiently update and render just the right components when your data changes
declarative views make your code more predictable and easier to debug
Virtual DOM¶
A virtual DOM is a lightweight JavaScript representation of the DOM, and updating it is faster than updating the real DOM
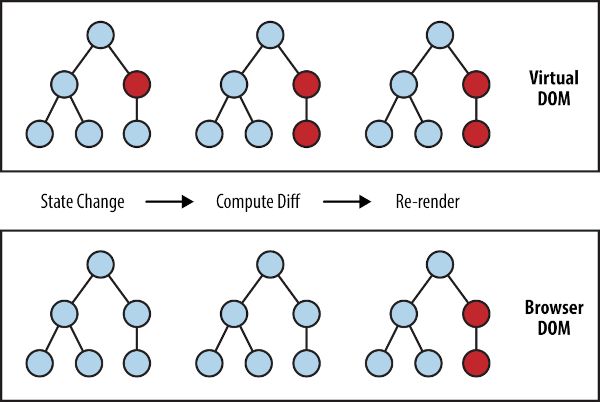
Virtual DOM and real browser DOM¶
When something inside a component changes:
the diff computations are done in the virtual DOM
ONLY the components (hierarchy) interested by that change will re-render.
This is one of the reason why React is so popular nowadays (2021)
Render method and JSX¶
React components implement a render() method that takes input data (props) and returns what to display.
It takes advantage of the XML-like syntax called JSX that allows to use html tags in javascript.
The JSX syntax allows also to use a special syntax ({someExpression}
) to write some JavaScript expressions in the html, and this creates a sort of template language.
In the example below react will create a tag h1
inside the html page, as child of div with id root
.
html page
<html>
<body>
<div id="root">
</body>
<html>
jsx code
const name = "John";
ReactDOM.render(
<h1>Hello {name + " " + name.toUpperCase()}</h1>,
document.getElementById('root')
);
will result in
<html>
<body>
<div id="root">
<h1>Hello John JOHN</h1>
</div>
</body>
<html>
Virtual DOM¶
A virtual DOM is a lightweight JavaScript representation of the DOM, and updating it is faster than updating the real DOM
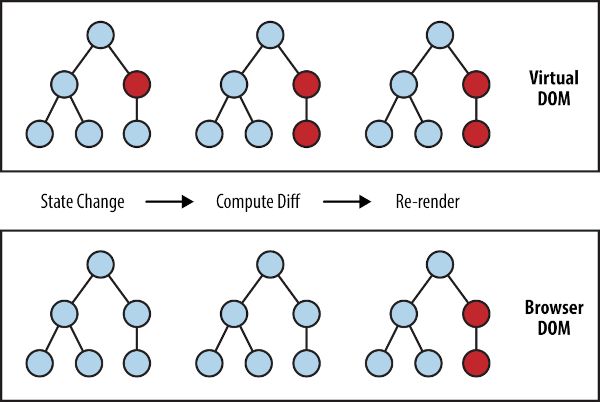
Virtual DOM and real browser DOM¶
When something inside a component changes:
the diff computations are done in the virtual DOM
ONLY the components (hierarchy) interested by that change will re-render.
This is one of the reason why React is so popular nowadays (2021)
Components¶
You can think of components as “new html tags” that can be used in JSX templates to represent HTML parts. Simple components can be used to compose bigger and more complex components.
Components can be defined by a class
or a function
.
In class components you will have the method render that return the effective React Element (that is defined by some JSX code):
class component
class Welcome extends React.Component {
render() {
return <h1>Hello, {this.props.name}</h1>;
}
}
in functional components (introduced in React 16.8) instead, the function returns directly the Element (JSX):
functional component
function Welcome(props) {
return <h1>Hello, {props.name}</h1>;
}
In both cases, components defined like that can be used in other components or rendered in the DOM.
ReactDOM.render(<Welcome name="Goku" />,
document.getElementById('root')
);
will result in
<html>
<body>
<div id="root">
<h1>Hello Goku</h1>
</div>
</body>
<html>
Note
Nowadays functional components are preferred. You can find in MapStore several class components developed before the introduction of some functionalities, like react hooks
React hooks¶
Hooks let you use state and other React features without writing a class. They are functions that let you “hook into” React state and lifecycle features from function components. Hooks don’t work inside classes
Here are the most common ones:
useState
useEffect
useRef
useState is used to store inside the component scope a local state, normally it is related to something that is not needed to be stored in the global state ans shared between various components of the app
function ExampleWithManyStates() {
// Declare multiple state variables!
const [age, setAge] = useState(42);
const [fruit, setFruit] = useState('banana');
const [todos, setTodos] = useState([{ text: 'Learn Hooks' }]);
// ...
}
useEffect is used to perform side effects in function components and replaces the functionalities usually done in lifecycle methods of the class components.
function Example() {
const [fruit, setFruit] = useState('banana');
// Similar to componentDidMount
useEffect(() => {
// run async task or initialize something or subscribe to some API
return () => {
// Similar to componentDidUnmount
// unsubscribe to Api or clean up things
}
}, []);
// Similar to componentDidMount
useEffect(() => {
// called whenever when the parameters passed change
}, [fruit]);
return <div> my favorite fruit is {fruit} </div>
}
If you want it to behave like componentDidMount
you have to pass it an empty array
If you want it to behave like componentWillUnmount
you have to return a function that react will call when he will do the clean up
Otherwise it will work as a normal componentDidUpdate lifecycle
Stateful component¶
A component can have its own state and when it changes the component re-renders.
Class components has their state accessible by this.state, and a method setState
to modify its value.
class Counter extends React.Component {
constructor(props) {
super(props);
this.state = { // here you can initialize the state
counter: 0
};
}
render() {
return (
<div>
<p>You clicked {this.state.counter} times</p>
<button onClick={() => {
// on button click, the counter is increased
this.setState({ counter: this.state.counter + 1 })
}}>
Click me
</button>
</div>
);
}
}
In functional components, the state (and other aspects of the original lifecycle methods implemented at the beginning in class components) has been
implemented using react hooks, in particular the state management is implemented by the hook useState
.
import React, { useState } from 'react';
function Counter() {
// Declares a new state variable, and get the value and the function to change it (setter).
const [counter, setCounter] = useState(0);
return (
<div>
<p>You clicked {counter} times</p>
<button onClick={() => setCounter(counter + 1)}>
Click me
</button>
</div>
);
}
In this case the state can be declared inside the functional component, with less code.
Note
the scope of this training is not to learn react, but only to have a general overview about the things you can see during the training. So we will not explore all the hooks, or the old lifecycle methods, but only see the general concept.
React Events¶
Handling events with React elements is very similar to handling events on DOM elements. There are some syntax differences:
React events are named using camelCase, rather than lowercase.
With JSX you pass a function as the event handler, rather than a string.
For example, the HTML:
<button onclick="activateLasers()">
Activate Lasers
</button>
function activateLasers() {}
<button onClick={activateLasers}>
Activate Lasers
</button>
Checkout here the list of the react events available here